Advanced Features
Cursor implements several advanced features that extend its functionality beyond standard code editors. These capabilities are designed to optimize the coding workflow and enhance code comprehension. The following sections detail key features that leverage AI and novel UI paradigms to augment the development process.
Cursor Predictions
Cursor Predictions is an AI-driven feature that generates context-aware code suggestions in real-time. This functionality extends beyond traditional autocomplete by offering multi-line, syntactically correct code snippets based on the current coding context. The system operates as follows:
- The AI model analyzes the current code context and historical patterns.
- It generates statistically likely continuations of the code.
- Suggestions are presented inline, allowing for immediate integration or dismissal.
- The model adapts to individual coding patterns over time, improving prediction accuracy.
This feature is particularly effective for:
- Rapid prototyping of code structures
- Reducing boilerplate code generation
- Suggesting implementations for unfamiliar APIs
- Maintaining consistent coding patterns across a project
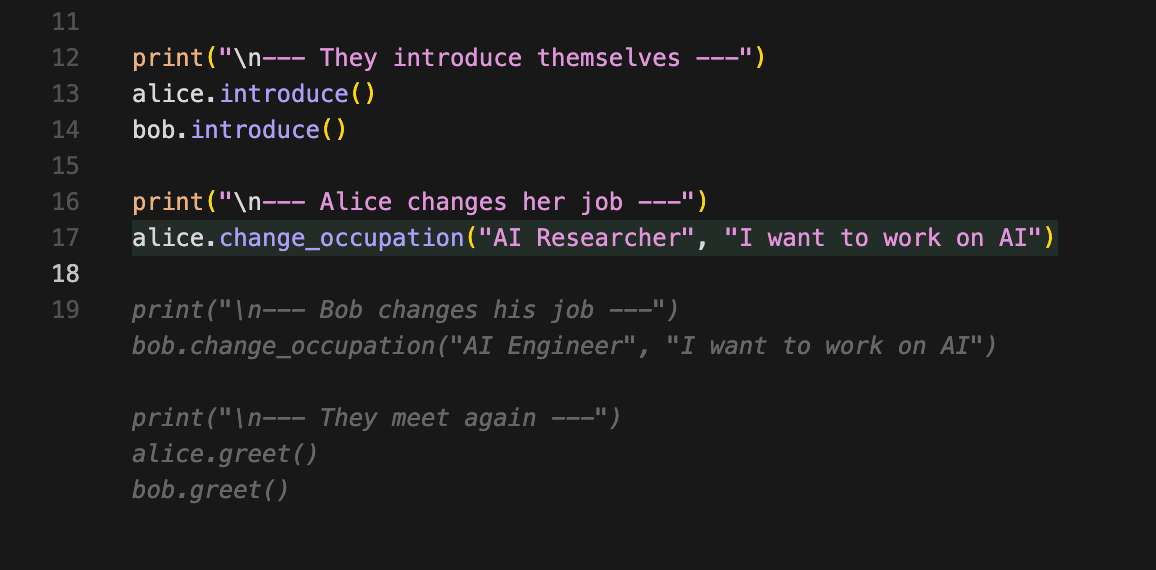
Figure 1: Cursor Predictions example, showing how AI generates context-aware code suggestions.
Peek and Edit
The Peek and Edit feature implements an inline code navigation and modification system. This functionality allows developers to view and edit code definitions without switching context or files. The mechanism operates as follows:
- Ctrl+Click on a symbol (function, class, or variable) activates the Peek view.
- "Go to Definition" or "Go to Type Definition" commands reveal the source of a symbol.
- The peeked code is editable directly within the current context.
- Modifications propagate immediately across the entire project.
This feature is particularly useful for:
- Efficient refactoring of code structures
- In-context debugging and code analysis
- Simultaneous interface and implementation modifications
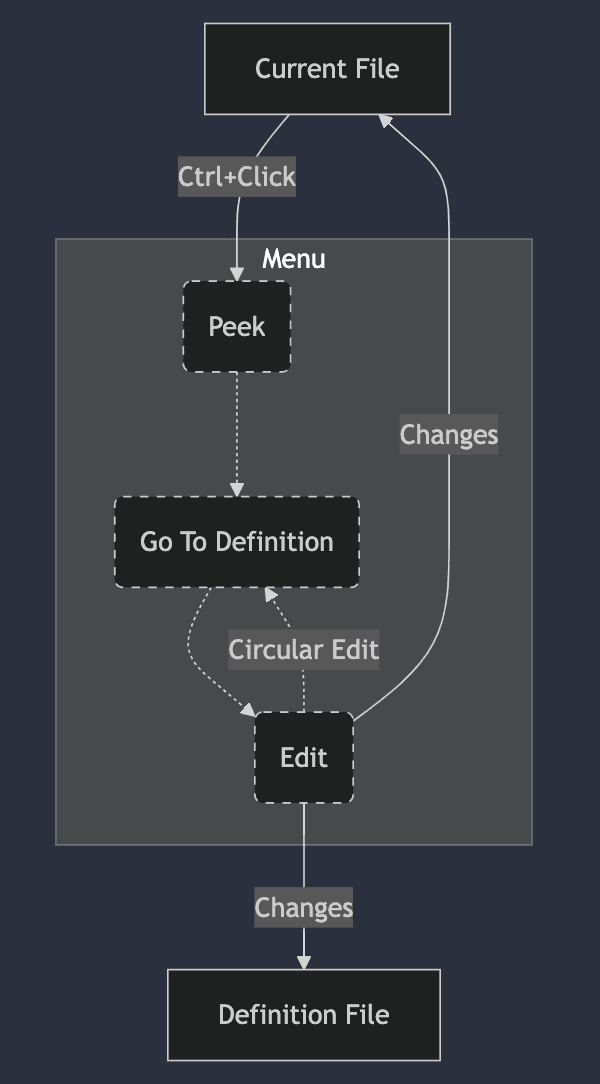
Figure 2: Cursor's Peek and Edit workflow, showing how changes in the peek view instantly propagate to all relevant files.
Example Workflow
Let's walk through a typical workflow using Cursor's Peek and Edit feature:
Say you have a class definition for a Person in a file called person.py.
class Person: def __init__(self, name: str, age: int, occupation: str): self.name = name self.age = age self.occupation = occupation def greet(self): print(f"Hello, I'm {self.name}!") def introduce(self): print(f"My name is {self.name}. I'm {self.age} years old and I work as a {self.occupation}.") def change_occupation(self, new_occupation: str): old_occupation = self.occupation self.occupation = new_occupation print(f"I've changed my job from {old_occupation} to {new_occupation}.")
And you also have a person interaction file that calls person.py called person_interaction.py
from person import Person # Create two Person instances alice = Person("Alice", 28, "Software Developer") bob = Person("Bob", 32, "Data Analyst") # Interaction between Alice and Bob print("--- Alice and Bob meet ---") alice.greet() bob.greet() print("\n--- They introduce themselves ---") alice.introduce() bob.introduce() print("\n--- Alice changes her job ---") alice.change_occupation("AI Researcher")
Say you want to add a reason for changing occupation in the interaction file. You can immediately change the method definition in the person.py file without leaving the file.
a. Hit Ctrl+Click on the change_occupation method in the interaction file:
b. Hit "Go to Definition" in the peek view:
c. Change the method implementation within the peek view and see the changes propagate (in this case, you can see Cursor auto suggested the reason for changing occupation):
This streamlined process eliminates the need for constant file switching, helping you maintain focus and improve productivity.
Partial Accepts
Partial Accepts is a feature that enables granular incorporation of AI-generated code suggestions. To activate this functionality:
- Access the Cursor IDE settings (typically via the gear icon or Ctrl+,).
- Navigate to the "Features" section in the settings menu.
- Locate the "Partial Accepts" option and set it to "enabled".
- Restart the Cursor IDE to apply the changes.
Once activated, Partial Accepts allows for selective integration of code suggestions:
- The system generates multi-line code suggestions based on the current context.
- Developers can review and selectively accept specific segments of the suggestion.
- Unwanted portions of the suggestion can be discarded.
- Accepted segments are seamlessly integrated into the existing codebase.
- To accept the next word of a suggestion, use Ctrl/⌘ + right arrow. This keybinding can be customized by modifying the
editor.action.inlineSuggest.acceptNextWord
setting.
This feature is particularly effective for:
- Selective implementation of complex algorithms or design patterns
- Granular application of AI-suggested code improvements
- Incremental learning and integration of new coding techniques
- Maintaining consistent code style while leveraging AI suggestions
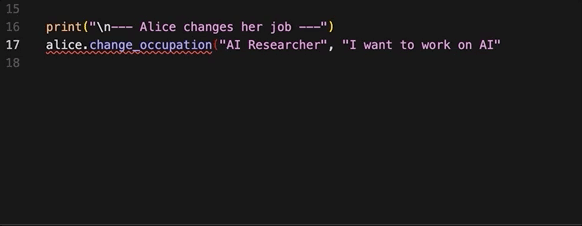
Figure 3: Partial Accepts example, demonstrating how developers can selectively incorporate AI-generated code suggestions.